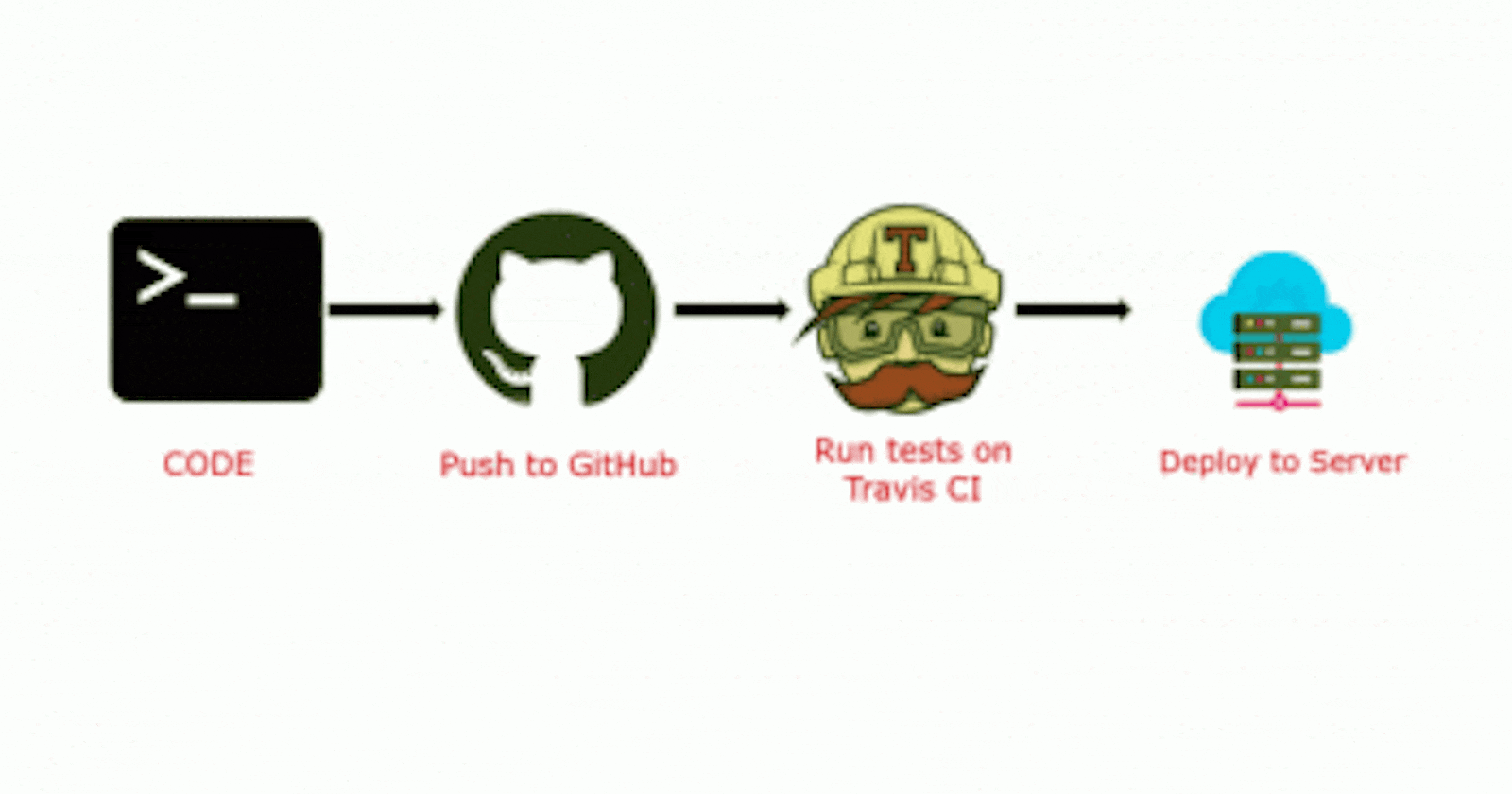
Django REST Framework - 2. Set up CI/CD with Travis CI, Github & Docker following Test Driven Development.
In our previous blog i.e. Django REST Framework - 1. Dockerize Your Project, we learned how to set up our project with Docker, Now we'll learn how to enable Travis CI for our Github Project. Travis is a Continuous Integration tool that automates our Python Unit tests and Linting every time we push our code to Github. So let's get started...
Firstly visit travis-ci.com and signup with your Github account. Once you signup, Go To Repositories. Now click on Manage repositories on Github and provide access to All repositories OR repositories of your choice. Once you are done, It will look something like this.
Now go to your project and follow the steps below.
Create Travis CI configuration file.
Create a new file named .travis.yml in the root of your project directory and enter the following commands as shown below.
Configure flake8.
Add flake8 requirements to your requirements.txt file.
Let's add flake8 config within our Django App. Create a new file ".flake8" and add the following exclusions.
Commit your changes and generate a build.
Once you commit and push your changes to Github. Take a look at your Travis page i.e. Our Travis project https://app.travis-ci.com You can see it started to build our project for our new changes.
deep@Latitude-5590:~/Desktop/DRF/DRF-Guide$ git add . deep@Latitude-5590:~/Desktop/DRF/DRF-Guide$ git commit -am 'ADDED: travis cl & flake8 configurations' [main 639789f] ADDED: travis cl & flake8 configurations 3 files changed, 26 insertions(+), 1 deletion(-) create mode 100644 .travis.yml create mode 100644 app/.flake8 deep@Latitude-5590:~/Desktop/DRF/DRF-Guide$ git push origin main Counting objects: 6, done. Delta compression using up to 8 threads. Compressing objects: 100% (6/6), done. Writing objects: 100% (6/6), 874 bytes | 874.00 KiB/s, done. Total 6 (delta 1), reused 0 (delta 0) remote: Resolving deltas: 100% (1/1), completed with 1 local object.
So this is how you configure Travis CI for your project.
Test-Driven Development.
Test-driven development is simply when you write your tests before you write your code. Let's go ahead and write our test cases.
Consider an example of a Calculator. We'll write a simple test to Add and Subtract two numbers. Go to your "tests.py" file in your app folder and add test cases as shown below.
from Django.test import TestCase # Create your tests here. class CalcTests(TestCase): def test_add_two_numbers(self): """ Test to add numbers """ self.assertEqual(add(1,5), 6) def test_sub_numbers(self): """ Test to subtract numbers """ self.assertEqual(subtract(5, 11), 6)
Now go to your terminal and run Unit tests using the command" docker-compose run app sh -c "python manage.py test" . Because we haven't written code for any of the above tests, the test cases will fail.
deep@Latitude-5590:~/Desktop/DRF/DRF-Guide$ docker-compose run app sh -c Creating test database for alias 'default'... System check identified no issues (0 silenced). ====================================================================== ERROR: test_add_numbers (myapp.tests.CalcTests) Test to add numbers ---------------------------------------------------------------------- Traceback (most recent call last): File "/app/myapp/tests.py", line 9, in test_add_numbers self.assertEqual(add(1,5), 6) NameError: name 'add' is not defined ====================================================================== ERROR: test_sub_numbers (myapp.tests.CalcTests) Test to subtract numbers ---------------------------------------------------------------------- Traceback (most recent call last): File "/app/myapp/tests.py", line 13, in test_sub_numbers self.assertEqual(subtract(5, 11), 6) NameError: name 'subtract' is not defined ---------------------------------------------------------------------- Ran 2 tests in 0.001s FAILED (errors=2) Destroying test database for alias 'default'...
As expected our tests failed. So let's go ahead and write our basic methods to Add and Subtract two numbers. Create a new file called utils.py and add code for Add and Subtract methods.
Import add & subtract methods we created in "utils.py" to our "tests.py " file as
from django.test import TestCase from myapp.utils import add, subtract
Now try running your test cases again. You will see your test cases have passed successfully.
deep@Latitude-5590:~/Desktop/DRF/DRF-Guide$ docker-compose run app sh -c "python manage.py test" Creating test database for alias 'default' System check identified no issues (0 silenced). .------------------------------------------------------ Ran 2 tests in 0.001s OK Destroying test database for alias 'default'...
Go ahead and commit your code to Github, Take a look at your Travis app. You'll see code is deployed successfully, passing our Test cases and flake8.
So, that's how you write tests and high-quality code, following TDD.
Thank you for reading...